Try Except Python
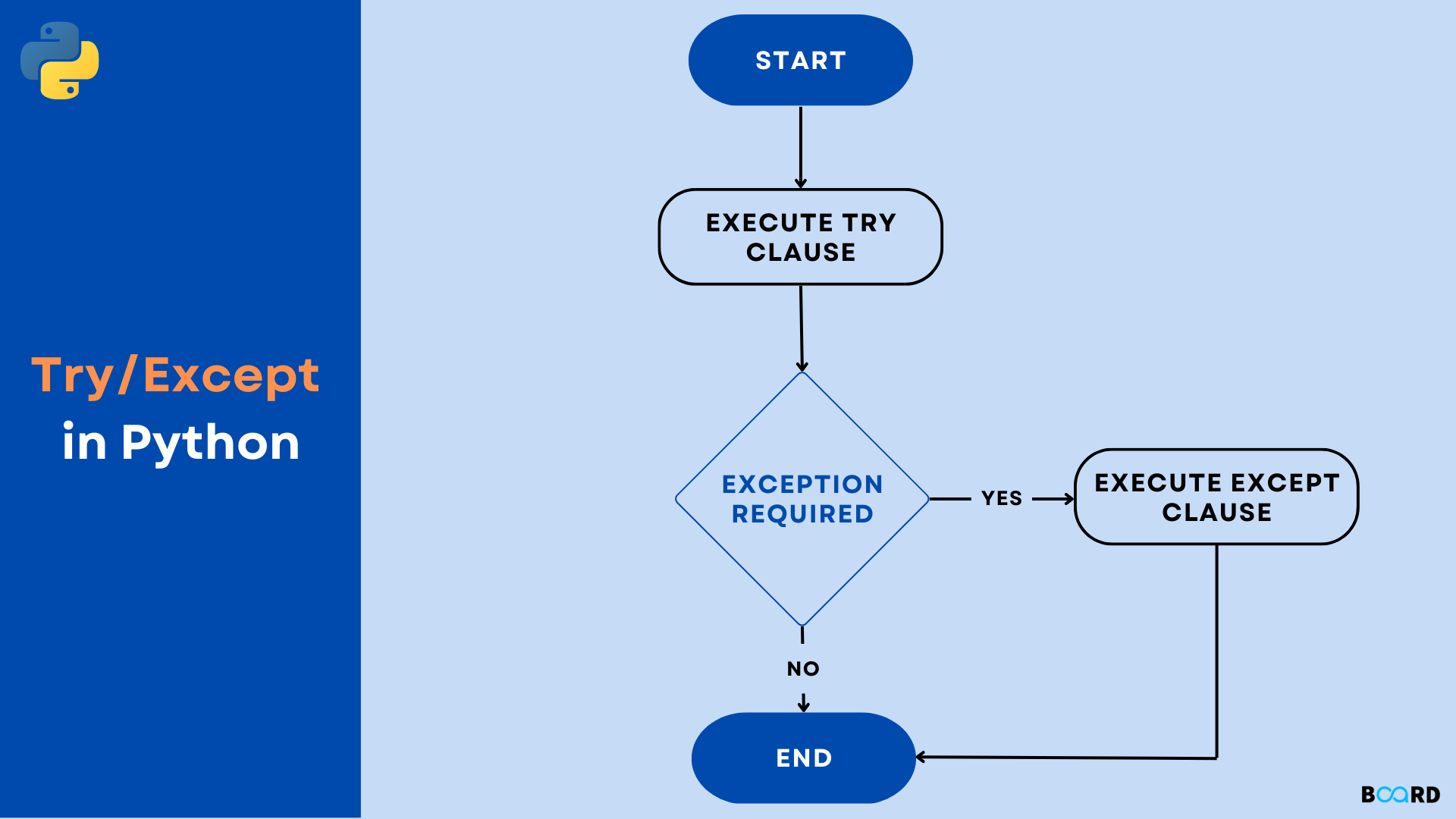
In the world of programming, errors are inevitable. No matter how carefully you write your code, there will always be unforeseen circumstances that can lead to unexpected behavior or failures. Python, being a versatile and widely-used programming language, offers robust mechanisms to handle errors effectively. One of the fundamental tools in Python’s error handling arsenal is the try-except
block.
Understanding the Basics
At its core, the try-except
block allows you to catch and handle exceptions that occur during the execution of your code. The syntax is straightforward:
try:
# Code block where an exception may occur
# ...
except ExceptionType:
# Code block to handle the exception
# ...
Here’s how it works: Python executes the code inside the try
block. If an exception occurs within this block, Python immediately jumps to the corresponding except
block. If no exception occurs, the except
block is skipped entirely.
Handling Specific Exceptions
Python allows you to catch specific types of exceptions by specifying the exception type after the except
keyword. This granularity gives you precise control over how different types of errors are handled. For example:
try:
# Code block where an exception may occur
# ...
except ValueError:
# Handle ValueError exceptions
# ...
except ZeroDivisionError:
# Handle ZeroDivisionError exceptions
# ...
except Exception as e:
# Handle any other exceptions
# ...
By catching specific exceptions, you can tailor your error handling strategy to different scenarios within your code.
The Exception Hierarchy
In Python, exceptions are organized in a hierarchy. At the top of this hierarchy is the BaseException
class, from which all other built-in exceptions inherit. This hierarchy allows you to catch broad categories of exceptions or specific types as needed. For example:
try:
# Code block where an exception may occur
# ...
except BaseException as e:
# Handle any exception
# ...
The else and finally Clauses
In addition to try
and except
, Python offers two optional clauses that further enhance the error handling capabilities: else
and finally
.
The else
clause is executed if the code block inside the try
block completes without raising any exceptions. This is useful for code that should only run if no exceptions occur. For example:
try:
# Code block where an exception may occur
# ...
except SomeException:
# Handle SomeException
# ...
else:
# Code to execute if no exception occurs
# ...
The finally
clause is always executed, regardless of whether an exception occurs. It is commonly used for cleanup tasks, such as closing files or releasing resources. For example:
try:
# Code block where an exception may occur
# ...
except SomeException:
# Handle SomeException
# ...
finally:
# Cleanup code that always executes
# ...
Common Use Cases
The try-except
block is invaluable in many real-world scenarios, including:
- File Handling: When reading from or writing to files, errors such as file not found or insufficient permissions may occur. Using
try-except
, you can gracefully handle these situations. - Network Operations: When making network requests, errors such as timeouts or connection errors are common. By wrapping network-related code in a
try-except
block, you can handle these errors without crashing your program. - User Input Validation: When writing interactive programs, validating user input is essential.
try-except
blocks can catch input that doesn’t meet specified criteria and prompt the user to try again. - Database Operations: When working with databases, errors like connection failures or SQL syntax errors can occur. Using
try-except
, you can handle these errors and provide meaningful feedback to the user.
Best Practices
While try-except
blocks are powerful tools for error handling, they should be used judiciously. Here are some best practices to keep in mind:
- Be Specific: Catch specific exceptions whenever possible to avoid inadvertently catching unrelated errors.
- Keep it Simple: Don’t overcomplicate error handling logic. Aim for clarity and readability.
- Avoid Bare Excepts: Avoid using bare
except
clauses (except:
) without specifying the exception type. This can catch unexpected errors and make debugging difficult. - Log Errors: Consider logging exceptions to aid in debugging and troubleshooting.
- Use Multiple Except Blocks: When handling different types of exceptions, use separate
except
blocks for each type to maintain clarity and organization.
Conclusion
In Python, the try-except
block is a powerful mechanism for handling errors gracefully. By anticipating potential exceptions and providing appropriate error-handling logic, you can write more robust and reliable code. Understanding how to effectively use try-except
blocks is an essential skill for any Python developer, enabling them to create software that is more resilient and user-friendly. So next time you encounter an error in your Python code, remember the try-except
block and wield its power to tame those exceptions.